Vert.x provides two ways to use traditional blocking APIs safely within a Vert.x application. One of these ways is the
executeBlocking
method, which executes the blocking code and specifies a result handler to be called back asynchronous when the blocking code has been executed.
In this way, you can run inline blocking code directly while on an event loop. By default, blocking code is executed on the Vert.x worker pool. Its configuration can be customized according to your needs through the
DeploymentOptions
object with the setWorkerPoolSize
. For example, if you set the worker pool size to 100, then Vert.x will use this pool to run blocking code. Also, Vert.x allows additional pools to be created for different purposes.
When it’s no longer necessary, the worker executor must be closed.
Running inline blocking code can be enough in some cases, but to take full advantage of the code encapsulate advantage and for clean code, in most cases, it would be more useful to use the worker verticles.
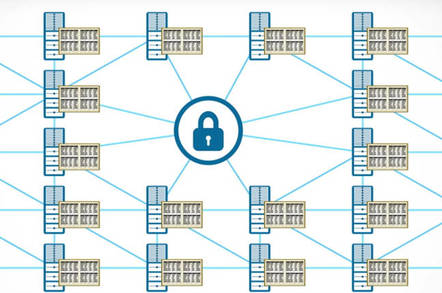
Worker Verticles
Worker Verticles are similar to standard verticles. The only difference is the use of a thread from the worker pool as distinct from the standard verticles. Hence, the worker verticles won't block any event loops.
To deploy a verticle as a worker you do set
setWorker
to true
through DeploymentOptions
.
That is all.
Conclusion
Vert.x doesn't want the event loop to be blocked because of its asynchronous architecture, but we usually work with synchronous APIs by necessarily in many cases. Fortunately, Vert.x provide two ways that they are inline, and the worker verticle uses traditional blocking APIs safely within a Vert.x application for these situations. For clean code and to take full advantage of the encapsulation, I think it is more accurate to use worker verticles in many cases.
No comments:
Post a Comment